Logging in users
Mobile API
The process of logging in a user with the Mobile API is similar to creating a new one, but the difference is you need to provide two credentials (phone number and birthdate) before calling the /login
endpoint.
Therefore, there are four steps in total to login a user:
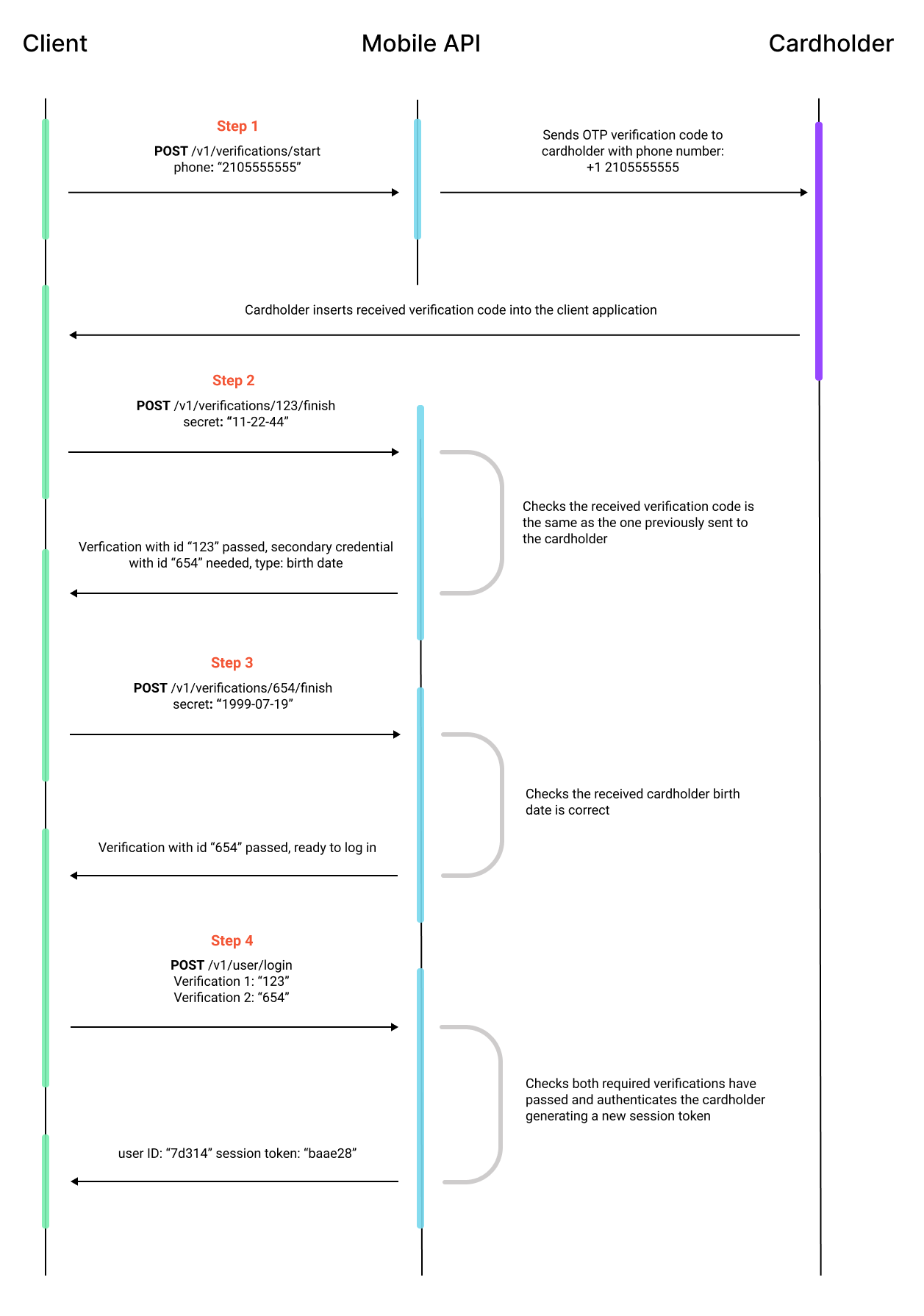
Step 1: Send a verification code to the user
Begin the current user verification process by sending a POST request to the /verifications/start
endpoint with the user's phone number (or email if email is the primary credential):
The phone number is validated using a google phonelib library. Email validation is done by checking if the domain exists and MX register is configured.
When testing your program in Sandbox, you'll only be able to create users with U.S. phone numbers. If you're a Blue or Orange customer and would like to use non-U.S. phone numbers to test cardholder operations, contact us at
- cURL
- JSON
{
"datapoint": {
"data_type": "phone",
"country_code": "1",
"phone_number": "2105555555"
},
"datapoint_type": "phone"
}
curl --location --request POST 'https://api.aptopayments.com/v1/verifications/start' \
--header 'Api-Key: Bearer {MOBILE_API_KEY}' \
--header 'Content-Type: application/json' \
--data-raw '{
"datapoint": {
"data_type": "phone",
"country_code": "1",
"phone_number": "2105555555"
},
"datapoint_type": "phone"
}'
When you submit this request, it triggers a 6 digit one-time password (OTP) via SMS to the user's phone number which expires after 15 minutes.
The response will be a verification object containing the verification process ID (verification_id
) and its status, which should be “pending” (as the process is not yet complete):
{
"type": "verification",
"verification_id": "entity_27288bc122db1339",
"status": "pending"
}
Step 2: Verify the user’s primary credential
Once the user receives the OTP, you can send a POST request with it to the the /verifications/{verification_id}/finish
endpoint to complete the verification. You will also need to send the verification process ID received in Step 1.
The OTP received by the user will include a dash (eg: 111-222
); however, when sending the request do not include the dashes (e.g. 111222
).
- cURL
- JSON
{
"secret": "111222"
}
curl --location --request POST 'https://api.aptopayments.com/v1/verifications/{VERIFICATION_ID}/finish' \
--header 'Api-Key: Bearer {MOBILE_API_KEY}' \
--header 'Content-Type: application/json' \
--data-raw '{
"secret": "111222"
}'
The response will include the status of the current verification process, which can be one of the following four values: pending
, passed
, failed
, expired
.
{
"type": "verification",
"verification_id": "entity_27288bc122db1339",
"status": "passed",
"secondary_credential": {
"verification_id": "entity_baae28af702d231f",
"type": "verification",
"verification_type": "birthdate",
"status": "pending"
}
}
Save the two verification_id
values of this payload, as you'll need them for the final API call to the /user/login
endpoint.
- 1st Verification ID → primary credential (in our case, the user’s phone)
- 2nd Verification ID → secondary credential (in our case, the user’s date of birth)
Step 3: Verify the user’s secondary credential
Now send another POST request to the same endpoint, the /verifications/{verification_id}/finish
endpoint.
For this call, use the secondary credential verification_id
obtained in the payload of the previous call.
Set the value of secret
within the request body to the user’s birth date, following this format: YYYY-MM-DD
:
- cURL
- JSON
{
"secret": "1999-07-19"
}
curl --location --request POST 'https://api.aptopayments.com/v1/verifications/{VERIFICATION_ID}/finish' \
--header 'Api-Key: Bearer {MOBILE_API_KEY}' \
--header 'Content-Type: application/json' \
--data-raw '{
"secret": "1999-07-19"
}'
If successful, the response will include the status of the second verification, which should now be passed
:
{
"type": "verification",
"verification_id": "entity_27288bc122db1339",
"status": "passed"
}
Step 4: Log in the user
Send a POST request to the /user/login
endpoint, passing the two “passed” verification IDs in the request body.
Remember, the first verification_id
corresponds to the user's phone number verification, and the second one to the user's date of birth verification:
- cURL
- JSON
{
"verifications": {
"type": "list",
"data": [
{
"verification_id": "entity_7d314c02e05c1221"
},
{
"verification_id": "entity_baae28af702d231f"
}
]
}
}
curl --location --request POST 'https://api.aptopayments.com/v1/user/login' \
--header 'Api-Key: Bearer {MOBILE_API_KEY}' \
--header 'Content-Type: application/json' \
--data-raw '{
"verifications": {
"type": "list",
"data": [
{
"verification_id": "entity_7d314c02e05c1221"
},
{
"verification_id": "entity_baae28af702d231f"
}
]
}
}'
If successful, the response will contain a Session Token (user_token
) that you can use for future cardholder operations.
{
"type": "user_id",
"user_id": "{USER_ID}",
"user_token": "{USER_SESSION_TOKEN}"
}
The default TTL (time to live) for the session token is 31,536,000 seconds (365 days). However, this can be configured based on each customer needs by contacting customer support.
Core API
Only Blue or Orange Programs can authenticate cardholders through the Core API.
Authenticating users with the Core API is done in a single request. This is in contrast to the Mobile API, which requires that you authenticate the user using 2FA to be able to secure a session token. Send a POST
request to the session endpoint with the ID of the user you intend to authenticate:
curl --location --request POST 'https://api.aptopayments.com/v1/partner/users/session' \
--header 'Authorization: Basic {CORE_API_KEY}' \
--header 'Content-Type: application/json' \
--data-raw '{
"user_id": "crdhldr_4dd83aa225ab9139756d"
}'
The response will contain the user ID and session token for the recently authenticated user:
{
"type": "user_id",
"user_id": "crdhldr_b04f29605a018f7d72a2",
"user_token": "E5PWX4T9dcPriIo...ao0QZLC6YL/dAyaA8"
}
SDKs
To authenticate users through our Mobile SDKs refer to the corresponding sections on their docs: